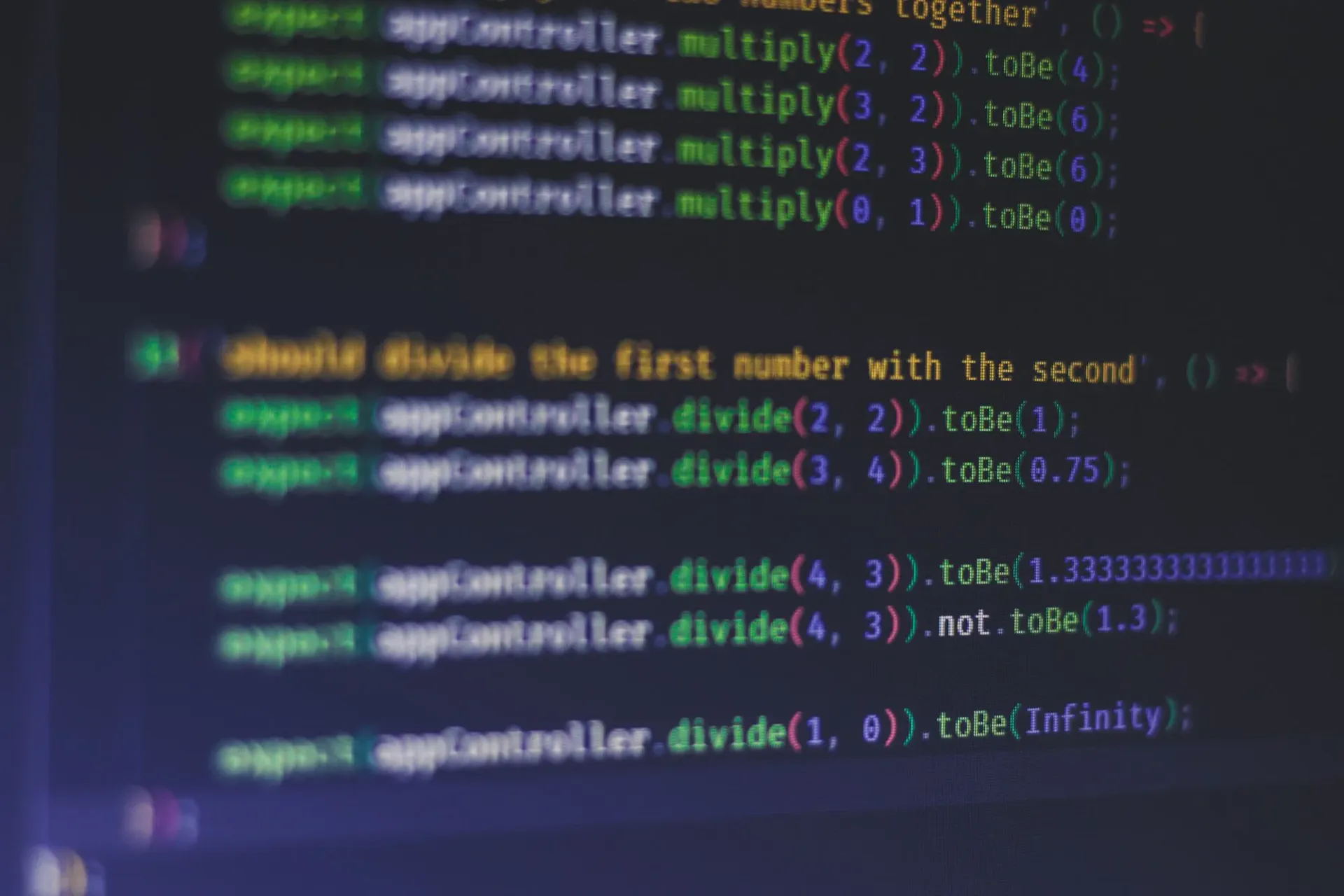
Introduction to Testing in JavaScript
Ensuring that your code behaves as expected, performs optimally, and remains maintainable with JavaScript testing.
Testing in JavaScript
Testing is a crucial part of the development process, ensuring that your code behaves as expected, performs optimally, and remains maintainable. In JavaScript, there are several types of testing methodologies, each serving a specific purpose in verifying different aspects of your code. The three main types of testing in JavaScript are unit testing, functional testing, and integration testing. Let's delve into each of these methodologies:
1. Unit Testing
What is Unit Testing?
Unit testing involves testing individual units or components of your code in isolation to ensure they perform as expected. A unit can be a function, method, or class. In JavaScript, unit tests are typically written using testing frameworks like Jest, Mocha, or Jasmine.
Key Characteristics of Unit Testing:
- Focuses on testing small, isolated units of code.
- Requires mocking or stubbing external dependencies to isolate the unit under test.
- Helps catch bugs early in the development process.
- Facilitates refactoring and code maintenance by providing a safety net.
Example:
Suppose you have a JavaScript function calculateTotal
that calculates the total price of items in a shopping cart. A unit test for this function would verify that it correctly calculates the total.
// Jest Example
test('calculateTotal calculates the total price correctly', () => {
expect(calculateTotal([10, 20, 30])).toBe(60);
});
2. Functional Testing
What is Functional Testing?
Functional testing, also known as black-box testing, evaluates the functionality of a software application by testing its user interface (UI) and user interactions. In JavaScript, functional testing is often performed using tools like Selenium, Cypress, or TestCafe.
Key Characteristics of Functional Testing:
- Focuses on testing the application from the end-user's perspective.
- Tests the application's features and interactions with external systems.
- Validates whether the application meets the specified requirements.
- Helps ensure the application behaves as expected across different browsers and devices.
Example: Consider a web application with a login feature. A functional test would simulate a user logging in and verify that the user is redirected to the correct page after successful authentication.
// Cypress Example
it('Successfully logs in user', () => {
cy.visit('/login');
cy.get('input[name="username"]').type('testuser');
cy.get('input[name="password"]').type('password');
cy.get('button[type="submit"]').click();
cy.url().should('include', '/dashboard');
});
3. Integration Testing
What is Integration Testing?
Integration testing verifies interactions between different components or modules of a system to ensure they work together correctly. In JavaScript, integration testing can be performed using testing frameworks like Jest, Mocha, or Supertest for backend applications.
Key Characteristics of Integration Testing:
- Tests how individual components interact and integrate with each other.
- Ensures that the system's modules communicate and exchange data as expected.
- Helps identify issues arising from the integration of multiple components.
- Validates the system's overall behavior and functionality.
Example:
Suppose you have an Express.js server with an endpoint /api/products
that retrieves a list of products from a database. An integration test would verify that the endpoint returns the expected data from the database.
// Jest + Supertest Example
test('GET /api/products returns list of products', async () => {
const response = await request(app).get('/api/products');
expect(response.status).toBe(200);
expect(response.body).toEqual(expect.arrayContaining([ /* Expected product data */ ]));
});
Conclusion
In summary, unit testing, functional testing, and integration testing are essential components of the software development lifecycle in JavaScript. By employing these testing methodologies effectively, you can ensure the reliability, functionality, and performance of your JavaScript applications.
For more in-depth information and examples on testing in JavaScript, refer to the documentation of your chosen testing frameworks and tools.